Q: What’s the object-oriented way to become wealthy?
A: Inheritance. 😒
Your vocabulary determines the reality of your life.
When two Python classes offer the same set of methods with different implementations, the classes are polymorphic and are said to have the same interface. An interface in this sense might involve a common inherited class and a set of overridden methods. This allows using the two objects in the same way regardless of their individual types. Python Cheat Sheet Tutorial. Python is a general-purpose interpreted, interactive, object-oriented, and high-level programming language. It was created by Guido van Rossum during 1985- 1990. Object-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects. In this tutorial, you’ll learn the basics of object-oriented programming in Python. Conceptually, objects are like the components of a system. Think of a program as a factory assembly line of sorts.
Python list comprehensions provide a concise way for creating lists. It consists of brackets containing an expression followed by a for clause, then zero or more for or if clauses: EXPRESSION for ITEM in LIST. The expressions can be anything - any kind of object can go into a list. A list comprehension always returns a list. Python For Data Science Cheat Sheet NumPy Basics Learn Python for Data Science Interactively at www.DataCamp.com NumPy DataCamp Learn Python for Data Science Interactively The NumPy library is the core library for scienti c computing in Python. It provides a high-performance multidimensional array object, and tools for working with these arrays.
In this tutorial, I have compiled the most essential terms and concepts of object-oriented programming in Python. My goal was to create the best Python OOP cheat sheet that shows them in one place.
Well — here it is:
Before we dive in the code from the Python OOP cheat sheet, let’s swipe through the most important OOP concepts in this Instagram post:
Ein Beitrag geteilt von The Python Blog (@finxter.com_) am
Free download 1 number one. Want to get more printable PDF cheat sheets like the following one?
Join my free email series with cheat sheets, free Python lessons, and continuous improvement in Python! It’s fun! 🙂
So let’s study the code!
Before we dive into the vocabulary, here’s an interactive Python shell:
Exercise: Create a new class Tiger that inherits from the parent class Cat and add a custom method!
Let’s dive into the Vocabulary!
OOP Terminology in Python

Class: A blueprint to create objects. It defines the data (attributes) and functionality (methods) of the objects. You can access both attributes and methods via the dot notation.
Object (=instance): A piece of encapsulated data with functionality in your Python program that is built according to a class definition. Often, an object corresponds to a thing in the real world. An example is the object Obama
that is created according to the class definition Person
. An object consists of an arbitrary number of attributes and methods, encapsulated within a single unit.
Instantiation: The process of creating an object of a class.
Method: A subset of the overall functionality of an object. The method is defined similarly to a function (using the keyword def
) in the class definition. An object can have an arbitrary number of methods.
Method overloading: You may want to define a method in a way so that there are multiple options to call it. For example for class X
, you define a method f(…)
that can be called in three ways: f(a)
, f(a,b)
, or f(a,b,c)
. To this end, you can define the method with default parameters (e.g., f(a, b=None, c=None)
).
Attribute: A variable defined for a class (class attribute) or for an object (instance attribute). You use attributes to package data into enclosed units (class or instance).
Class attribute (=class variable, static variable, static attribute): A variable that is created statically in the class definition and that is shared by all class objects.
Dynamic attribute: An “–>instance attribute” that is defined dynamically during the execution of the program and that is not defined within any method. Affiliate marketing success tips. For example, you can simply add a new attribute neew
to any object o by calling o.neew = ..
.
Instance attribute (=instance variable): A variable that holds data that belongs only to a single instance. Other instances do not share this variable (in contrast to “–>class attributes”). In most cases, you create an instance attribute x
in the constructor when creating the instance itself using the self
keywords (e.g., self.x = ..
).
Inheritance: Class A
can inherit certain characteristics (like attributes or methods) from class B
. For example, the class Dog
may inherit the attribute number_of_legs
from the class Animal
. In this case, you would define the inherited class Dog
as follows: class Dog(Animal): ..
Encapsulation: Binding together data and functionality that manipulates the data.

Python Oop Cheat Sheet Examples
If you have understood these OOP terms, you can follow most of the discussions about object-oriented programming. That’s the first step towards proficiency in Python!
Automate Your Learning Progress in Python
Thanks for reading that far—you’re clearly ambitious in mastering the Python programming language.
For your convenience, I have created a Python cheat sheet email series where you will get tons of free stuff (cheat sheets, PDFs, lessons, code contests). Join 5,253 subscribers. It’s fun!
Where to Go From Here?
Enough theory, let’s get some practice!
To become successful in coding, you need to get out there and solve real problems for real people. That’s how you can become a six-figure earner easily. And that’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
Practice projects is how you sharpen your saw in coding!
Do you want to become a code master by focusing on practical code projects that actually earn you money and solve problems for people?
Then become a Python freelance developer! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
Join my free webinar “How to Build Your High-Income Skill Python” and watch how I grew my coding business online and how you can, too—from the comfort of your own home.
Related Articles:
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com. He’s author of the popular programming book Python One-Liners (NoStarch 2020), coauthor of the Coffee Break Python series of self-published books, computer science enthusiast, freelancer, and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
Related Posts
Class inheritance is a very useful Object-oriented Programming construct for sharing and reusing code. Inheritance makes it possible to break up and organize your code into a hierarchy, from generic to specific. Objects that belong to classes that are higher up in the hierarchy (more generic) are accessible by subclasses, but not vice versa.
Earlier, we saw that bool
is a subclass of int
, thus, it inherited the properties and methods of the int
class, and then extended it to be more specific to booleans.
We can do the same with our own classes, too. In a file called vehicle.py
, let’s create a parent Vehicle
class, and have our Car
class be a subclass.
When we instantiate a Car instance, the interpreter calls __init__()
, where we pass in two arguments (make
and model
) and an optional 3rd (fuel
, which defaults to “gas”). In __init__()
, we call super().__init__()
, which resolves to our parent class, Vehicle
, and runs its__init__
function, where the variables are stored. Note that even though the variables are stored at the Vehicle
level, they are instance variables because self
is bound to my_car
, which is a Car
, which is a Vehicle
. Don’t forget to import your Vehicle and Car classes. Behold:
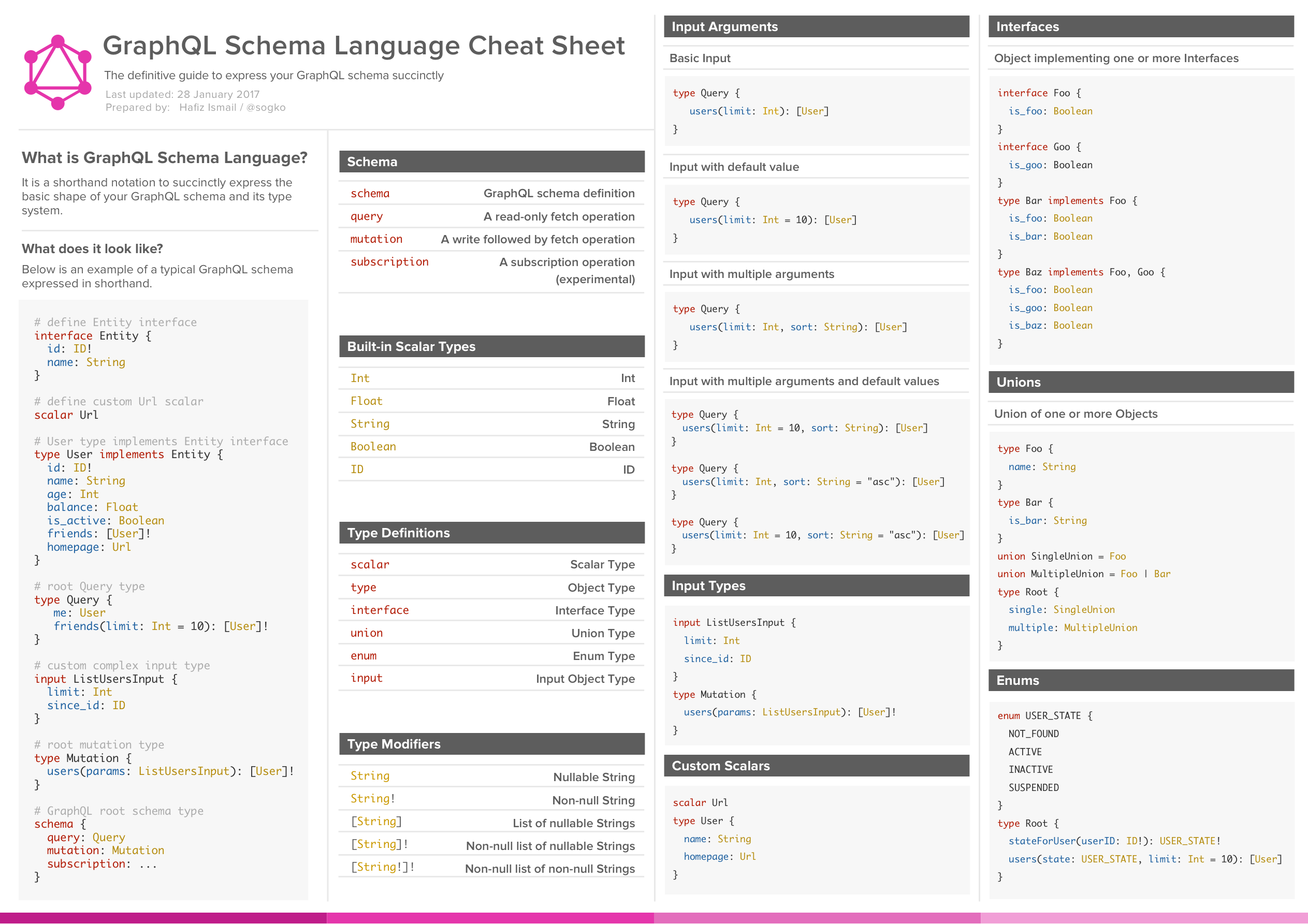
Overriding Variables in a Subclass
We can, of course, use a subclass to override variables that belong to a parent class. Let’s update our vehicle.py
file:
Note how our Truck’s class variable number_of_wheels
overrode the parent class Vehicle
’s number_of_wheels
(or, to be more specific, the interpreter found number_of_wheels
in a closer scope, the Truck
class, and did not need to continue searching up the hierarchy). Likewise, Motorcycle
overrides just the number_of_wheels
Aboutmadden nfl 16 download. variable to equal 2. Notice there is no __init__()
function in Motorcycle
- the number_of_wheels
variable is overridden but instantiating a Motorcycle just goes straight to the Vehicle.__init__()
method.

Multiple Inheritance in Python
Python Cheat Sheet Pdf Basics
Can Python classes inherit from multiple parent classes? Yes, this is called multiple inheritance. It’s not as commonly used for simple programs, but you’ll see it more often as you start using libraries.
One common use case for multiple inheritance in Python is for a type of class called a Mixin. Mixin classes tend to be used to quickly and easily add additional properties and methods into a class. This type of design pattern encourages code with composable architecture.
Python Oop Cheat Sheet Download
Unfortunately, we won’t have time to cover the topic of Multiple inheritance in this workshop… because it’s out of scope. 🤣
